Polygon
Polygon Parameters#
Parameters - as mentioned in Azarzadvillas Documentation - are:
-
Constructor : -
Polygon
-
*vertices
: All the vertices defining the polygon- numpy array of dimension 3 (ex:
(1,1,0)
)
- numpy array of dimension 3 (ex:
Polygon - Pentagon#
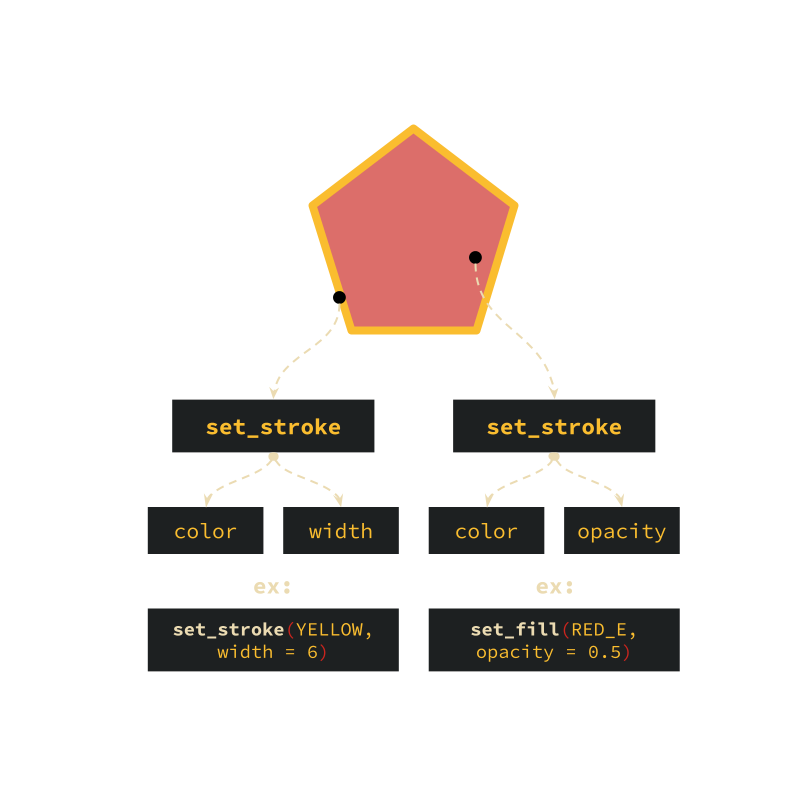
from manimlib import *
import numpy as np
class PentagonCreation(Scene):
def construct(self):
pentagon = [(0, 0, 0), (2, 0, 0),(2.7, 1.9, 0), (1, 3, 0),(-0.7, 1.9, 0)]
poly = Polygon(*pentagon)
# Yellow stroke
poly.set_stroke(YELLOW)
self.play(ShowCreation(poly), run_time=3)
self.wait()
#GREEN fill
poly.set_fill(GREEN, opacity=1)
self.wait()
return self
from manim import *
import numpy as np
class PentagonCreation(Scene):
def construct(self):
pentagon = [(0, 0, 0), (2, 0, 0),(2.7, 1.9, 0), (1, 3, 0),(-0.7, 1.9, 0)]
poly = Polygon(*pentagon)
# Yellow stroke
poly.set_stroke(YELLOW)
self.play(Create(poly), run_time=3)
self.wait()
#GREEN fill
poly.set_fill(GREEN, opacity=1)
self.wait()
return self
Polygon - Hexagon#
from manimlib import *
import numpy as np
class HexagonCreation(Scene):
def construct(self):
Hexagon = [(0, 0, 0), (2, 0, 0), (3, 1.8, 0), (2, 3.5, 0), (0, 3.5, 0), (-1.1, 1.8, 0)]
poly = Polygon(*Hexagon)
poly.set_stroke(YELLOW)
self.play(Create(poly), run_time=3)
poly.set_fill(GREEN, opacity=1)
self.wait()
return self
from manim import *
import numpy as np
class HexagonCreation(Scene):
def construct(self):
Hexagon = [(0, 0, 0), (2, 0, 0), (3, 1.8, 0), (2, 3.5, 0), (0, 3.5, 0), (-1.1, 1.8, 0)]
poly = Polygon(*Hexagon)
poly.set_stroke(YELLOW)
self.play(Create(poly), run_time=3)
poly.set_fill(GREEN, opacity=1)
self.wait()
return self
Polygon - Equilateral Triangle#
from manimlib import *
import numpy as np
class EquilateralTriangle(Scene):
def construct(self):
EqTri = [(0, 0, 0),(3, 0, 0),(1.5, 2.5, 0)]
poly = Polygon(*EqTri)
poly.set_stroke(YELLOW)
self.play(ShowCreation(poly), run_time=3)
poly.set_fill(GREEN, opacity=1)
self.wait()
return self
from manim import *
import numpy as np
class EquilateralTriangle(Scene):
def construct(self):
EqTri = [(0, 0, 0),(3, 0, 0),(1.5, 2.5, 0)]
poly = Polygon(*EqTri)
poly.set_stroke(YELLOW)
self.play(Create(poly), run_time=3)
poly.set_fill(GREEN, opacity=1)
self.wait()
return self
Polygon - Rectangle#
from manimlib import *
import numpy as np
class RecangleCreation(Scene):
def construct(self):
rec = [(0, 0, 0), (3, 0, 0), (3, 2, 0), (0, 2, 0)]
poly = Polygon(*rec)
poly.set_stroke(YELLOW)
self.wait()
self.play(ShowCreation(poly), run_time=3)
poly.set_fill(GREEN, opacity=1)
self.wait(2)
return self
from manim import *
import numpy as np
class RecangleCreation(Scene):
def construct(self):
rec = [(0, 0, 0), (3, 0, 0), (3, 2, 0), (0, 2, 0)]
poly = Polygon(*rec)
poly.set_stroke(YELLOW)
self.wait()
self.play(Create(poly), run_time=3)
poly.set_fill(GREEN, opacity=1)
self.wait(2)
return self