Circle
Circle Parameters#
Paremeters - as mentioned in Azarzadvillas Documentation - are:
Circle inherits from Arc
-
Constructor : -
Circle
-
radius
: Distance from arc_center to the arc- float (ex:
2
) - Optional
- Default:
1.0
- float (ex:
-
arc_center
: Point to which all points in the arc are equidistant- numpy array of dimension 3 (ex:
(1,1,0)
) - Optional
- Default:
ORIGIN
- numpy array of dimension 3 (ex:
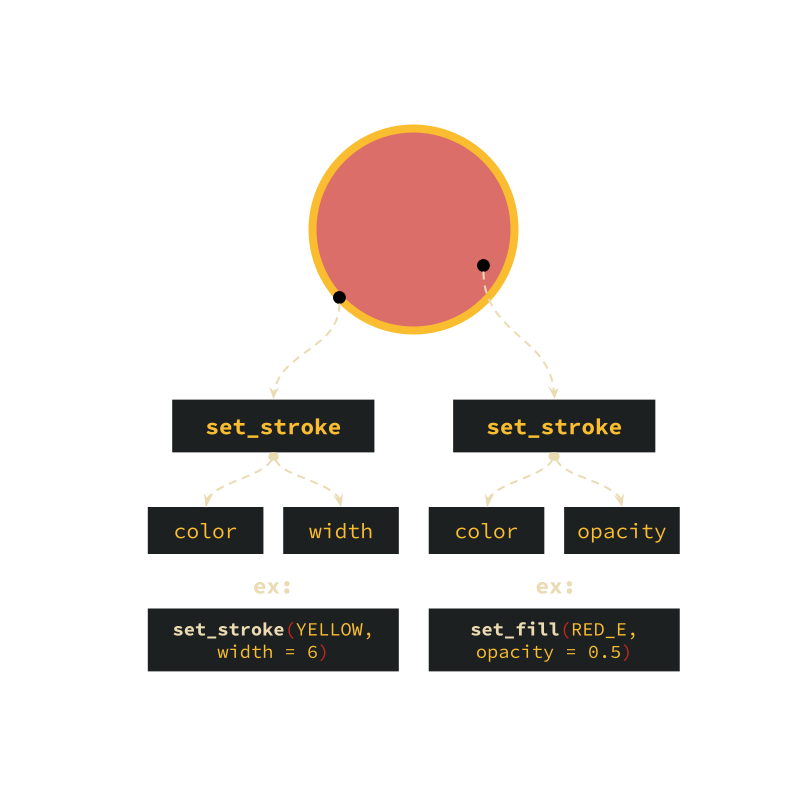
Add a circle#
from manimlib import *
class circle1(Scene):
def construct(self):
circle = Circle()
self.add(circle)
from manim import *
class circle1(Scene):
def construct(self):
circle = Circle()
self.add(circle)
Set stroke color & stroke width#
from manimlib import *
class circle1(Scene):
def construct(self):
circle = Circle()
circle.set_stroke(RED, width = 3)
self.add(circle)
from manim import *
class circle1(Scene):
def construct(self):
circle = Circle()
circle.set_stroke(RED, width = 3)
self.add(circle)
Set stroke color & fill color#
from manimlib import *
class circle1(Scene):
def construct(self):
circle = Circle()
circle.set_stroke(RED, width = 3)
circle.set_fill(YELLOW, opacity = 0.5)
self.add(circle)
from manim import *
class circle1(Scene):
def construct(self):
circle = Circle()
circle.set_stroke(RED, width = 3)
self.add(circle)
Draw a circle#
from manimlib import *
class circle1(Scene):
def construct(self):
circle = Circle()
circle.set_stroke(YELLOW_D, width = 3)
self.play(ShowCreation(circle))
circle.set_fill(YELLOW, opacity = 0.5)
self.wait()
from manim import *
class circle1(Scene):
Draw a circle in 3 seconds#
from manimlib import *
class circle1(Scene):
def construct(self):
circle = Circle()
# 3 represents the stroke width
circle.set_stroke(RED, 3)
# 0.5 represents the opacity of the fill
self.play(ShowCreate(circle), run_time=3)
circle.set_fill(RED_E, 0.5)
self.wait()
from manim import *
class circle1(Scene):
Fade a circle#
from manimlib import *
class circle1(Scene):
def construct(self):
circle = Circle()
circle.set_stroke(RED, 3)
self.play(ShowCreate(circle))
circle.set_fill(YELLOW, 0.5)
self.wait()
# run_time = x can also be added to play(FadeIn())
self.play(FadeIn(circle))
self.wait()
from manim import *
class circle1(Scene):
Remove a circle#
from manimlib import *
class circle1(Scene):
def construct(self):
circle = Circle()
circle.set_stroke(RED, 3)
self.play(ShowCreate(circle))
circle.set_fill(YELLOW, 0.5)
self.wait()
# run_time = x can also be added to play(FadeIn())
self.play(FadeIn(circle))
self.wait()
self.remove(circle)
from manim import *
class circle1(Scene):